一、循环结构
1.while循环结构
作用:满足循环条件,执行循环语句
语法:while(循环条件){循环语句}
解释:只要满足循环条件即执行循环语句,直到条件不符合时才停止
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| #include<iostream> using namespace std;
int main() { int n=0; while(n<10) { n++; cout<<n<<endl; }
return 0; }
|
2.do…while循环语句
作用:先执行一次循环语句,再判断循环条件
语法:do{循环语句} while(循环条件);
注意:与while循环语句的主要区别就是第一次判断的先后
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| #include<iostream> using namespace std;
int main() { int n=0; do { n++; cout<<n<<endl; } while(n>10);
return 0; }
|
3.for循环语句
语法:for(起始表达式;条件表达式;末尾循环体){循环语句;}
示例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| #include<iostream> using namespace std;
int main() { for(int n=0;n<10;n++) { cout<<n<<endl; }
return 0; }
|
4.嵌套循环
作用:在循环体中再嵌套一层循环,解决一些实际问题
示例:
打印*阵图
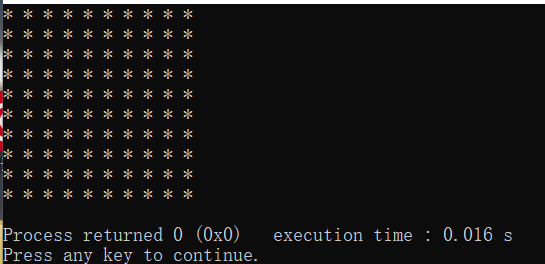
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| #include<iostream> using namespace std;
int main() { for(int i=0;i<10;i++) { for(int j=0;j<10;j++) { cout<<"* "; } cout<<endl; }
return 0; }
|
二、跳转语句
1.break语句
作用:用于跳出选择结构或者循环结构
break的使用时机
- 出现在switch条件语句中,作用是终止case并跳出switch
- 出现在循环语句中,作用是跳出当前循环语句
- 出现在嵌套中,作用是跳出最近的内层嵌套语句
2.continue语句
作用:在循环语句中,跳过本次循环中余下尚未执行的语句,继续执行下一次循环
示例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| #include<iostream> using namespace std;
int main() { for(int i=0;i<10;i++) { if(i%2==0) { continue; } cout<<i<<endl; } return 0; }
|
3.goto语句
作用:无条件跳转语句
语法:goto 标记;
解释:如果标记的名称存在的,执行goto语句时,会跳转到标记位置。(但是一般不用)
例如:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| #include<iostream> using namespace std;
int main() { cout<<"1"<<endl; goto flag; cout<<"2"<<endl; cout<<"3"<<endl; flag: cout<<"4"<<endl; return 0; }
|